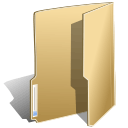
Font (5)
Retrieving and replacing fonts in Word documents is a key aspect of document design. This process enables users to refresh their text with modern typography, improving both appearance and readability. Mastering font adjustments can enhance the overall impact of your documents, making them more engaging and accessible.
In this article, you will learn how to retrieve and replace fonts in a Word document using Spire.Doc for Java.
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>13.6.2</version> </dependency> </dependencies>
Retrieve Fonts Used in a Word Document
To retrieve font information from a Word document, you'll need to navigate through the document's sections, paragraphs, and their child objects. For each child object, check if it is an instance of TextRange. If a TextRange is detected, you can extract the font details, including the font name and size, using the methods under the TextRange class.
Here are the steps to retrieve font information from a Word document using Java:
- Create a Document object.
- Load the Word document using the Document.loadFromFile() method.
- Iterate through each section, paragraph, and child object.
- For each child object, check if it is an instance of TextRange class.
- If it is, retrieve the font name and size using the TextRange.getCharacterFormat().getFontName() and TextRange.getCharacterFormat().getFontSize() methods.
- Java
import com.spire.doc.*; import com.spire.doc.documents.*; import com.spire.doc.fields.TextRange; import java.io.BufferedWriter; import java.io.FileWriter; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; // Customize a FontInfo class to help store font information class FontInfo { private String name; private Float size; public FontInfo() { this.name = ""; this.size = null; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Float getSize() { return size; } public void setSize(Float size) { this.size = size; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (!(obj instanceof FontInfo)) return false; FontInfo other = (FontInfo) obj; return name.equals(other.getName()) && size.equals(other.getSize()); } } public class RetrieveFonts { // Function to write string to a txt file public static void writeAllText(String filename, List<String> text) { try (BufferedWriter writer = new BufferedWriter(new FileWriter(filename))) { for (String s : text) { writer.write(s); } } catch (IOException e) { e.printStackTrace(); } } public static void main(String[] args) { List<FontInfo> fontInfos = new ArrayList<>(); StringBuilder fontInformations = new StringBuilder(); // Create a Document instance Document document = new Document(); // Load a Word document document.loadFromFile("C:\\Users\\Administrator\\Desktop\\input.docx"); // Iterate through the sections for (int i = 0; i < document.getSections().getCount(); i++) { Section section = document.getSections().get(i); // Iterate through the paragraphs for (int j = 0; j < section.getBody().getParagraphs().getCount(); j++) { Paragraph paragraph = section.getBody().getParagraphs().get(j); // Iterate through the child objects for (int k = 0; k < paragraph.getChildObjects().getCount(); k++) { DocumentObject obj = paragraph.getChildObjects().get(k); if (obj instanceof TextRange) { TextRange txtRange = (TextRange) obj; // Get the font name and size String fontName = txtRange.getCharacterFormat().getFontName(); Float fontSize = txtRange.getCharacterFormat().getFontSize(); String textColor = txtRange.getCharacterFormat().getTextColor().toString(); // Store the font information FontInfo fontInfo = new FontInfo(); fontInfo.setName(fontName); fontInfo.setSize(fontSize); if (!fontInfos.contains(fontInfo)) { fontInfos.add(fontInfo); String str = String.format("Font Name: %s, Size: %.2f, Color: %s%n", fontInfo.getName(), fontInfo.getSize(), textColor); fontInformations.append(str); } } } } } // Write font information to a txt file writeAllText("output/GetFonts.txt", Arrays.asList(fontInformations.toString().split("\n"))); // Dispose resources document.dispose(); } }
Replace a Specific Font with Another in Word
Once you obtain the font name of a specific text range, you can easily replace it with a different font, by using the TextRange.getCharacterFormat().setFontName() method. Additionally, you can adjust the font size and text color using the appropriate methods in the TextRange class.
Here are the steps to replace a specific font in a Word document using Java:
- Create a Document object.
- Load the Word document using the Document.loadFromFile() method.
- Iterate through each section, paragraph, and child object.
- For each child object, check if it is an instance of TextRange class.
- If it is, get the font name using the TextRange.getCharacterFormat().getFontName() method.
- Check if the font name is the specified font.
- If it is, set a new font name for the text range using the TextRange.getCharacterFormat().setFontName() method.
- Save the document to a different Word file using the Document.saveToFile() method.
- Java
import com.spire.doc.*; import com.spire.doc.documents.*; import com.spire.doc.fields.TextRange; public class ReplaceFont { public static void main(String[] args) { // Create a Document instance Document document = new Document(); // Load a Word document document.loadFromFile("C:\\Users\\Administrator\\Desktop\\input.docx"); // Iterate through the sections for (int i = 0; i < document.getSections().getCount(); i++) { // Get a specific section Section section = document.getSections().get(i); // Iterate through the paragraphs for (int j = 0; j < section.getBody().getParagraphs().getCount(); j++) { // Get a specific paragraph Paragraph paragraph = section.getBody().getParagraphs().get(j); // Iterate through the child objects for (int k = 0; k < paragraph.getChildObjects().getCount(); k++) { // Get a specific child object DocumentObject obj = paragraph.getChildObjects().get(k); // Determine if a child object is a TextRange if (obj instanceof TextRange) { // Get a specific text range TextRange txtRange = (TextRange) obj; // Get the font name String fontName = txtRange.getCharacterFormat().getFontName(); // Determine if the font name is Microsoft JhengHei if ("Microsoft JhengHei".equals(fontName)) { // Replace the font with another font txtRange.getCharacterFormat().setFontName("Segoe Print"); } } } } } // Save the document to a different file document.saveToFile("output/ReplaceFonts.docx", FileFormat.Docx); // Dispose resources document.dispose(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
When it comes to creating professional documents, choosing the right font is crucial. Using multiple fonts in one document can help distinguish different types of content such as headings, body text or annotations, ultimately enhancing the document's readability. Moreover, different fonts have unique emotional tones and styles. For instance, handwritten fonts often convey warmth and intimacy while serif fonts are ideal for traditional and formal contexts.
In this article, you will learn how to set the font in a Word document in Java using Spire.Doc for Java.
- Apply a Font to a Paragraph in Java
- Apply Multiple Fonts to a Paragraph in Java
- Use a Private Font in a Word Document in Java
- Change the Font of the Specified Text in Java
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>13.6.2</version> </dependency> </dependencies>
Apply a Font to a Paragraph in Java
Typically, a consistent font style throughout a paragraph keeps the text looking neat. Spire.Doc for Java provides the ParagraphStyle class, allowing you to define the font name, size, style, and text color using a single object. After creating a style, you can easily apply it to format a paragraph according to your preferred settings.
The steps to set the font for a paragraph using Java are as follows:
- Create a Document object.
- Load a Word file, and add a paragraph to it.
- Append text to the paragraph.
- Create a ParagraphStyle object.
- Get the CharacterFormat object using the ParagraphStyle.getCharacterFormat() method.
- Set the font style, name, size and text color using the methods under the CharacterFormat object.
- Add the style to the document using the Document.getStyles().add() method.
- Apply the defined style to the paragraph using the Paragraph.applyStyle() method.
- Save the document to a different Word file.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.Section; import com.spire.doc.documents.Paragraph; import com.spire.doc.documents.ParagraphStyle; import java.awt.*; public class ApplyFontToParagraph { public static void main(String[] args) { // Create a Document instance Document document = new Document(); // Load a Word document document.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx"); // Get the last section Section section = document.getLastSection(); // Add a paragraph Paragraph paragraph = section.addParagraph(); // Append text to the paragraph paragraph.appendText("Java's versatility and wide range of applications make it one of the most popular " + "programming languages in the world. Its strong community support and continuous evolution ensure " + "it remains relevant in modern software development."); // Create a ParagraphStyle object ParagraphStyle style = new ParagraphStyle(document); // Set the style name style.setName("MyStyle"); // Set the font style, name, size and text color style.getCharacterFormat().setBold(false); style.getCharacterFormat().setTextColor(Color.blue); style.getCharacterFormat().setFontName("Times New Roman"); style.getCharacterFormat().setFontSize(13f); // Add the style to document document.getStyles().add(style); // Apply the style to the paragraph paragraph.applyStyle(style.getName()); // Apply the style to an existing paragraph // section.getParagraphs().get(1).applyStyle(style.getName()); // Save the document to file document.saveToFile("output/ApplyFont.docx", FileFormat.Docx); // Dispose resources document.dispose(); } }
Apply Multiple Fonts to a Paragraph in Java
In certain situations, you might want to apply different fonts to various parts of the same paragraph to highlight important information. Spire.Doc for Java offers the TextRange class, which enables you to assign distinct styles to specific segments of text within a paragraph. To achieve this, ensure that the text requiring different styles is organized into separate text ranges.
The steps to apply multiple fonts in a paragraph using Java are as follows:
- Create a Document object.
- Load a Word file, and add a paragraph to it.
- Append text to the paragraph using the Paragraph.appendText() method, which returns a TextRange object.
- Append more text that needs to be styled differently to the paragraph and return different TextRange objects.
- Create a ParagraphStyle object with the basic font information and apply it to the paragraph.
- Change the font name, style, size and text color of the specified text range using the methods under the specific TextRange object.
- Save the document to a different Word file.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.Section; import com.spire.doc.documents.HorizontalAlignment; import com.spire.doc.documents.Paragraph; import com.spire.doc.documents.ParagraphStyle; import com.spire.doc.fields.TextRange; import java.awt.*; public class ApplyMultipleFonts { public static void main(String[] args) { // Create a Document object Document document = new Document(); // Load a Word document document.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx"); // Get the last section Section section = document.getLastSection(); // Add a paragraph Paragraph paragraph = section.addParagraph(); // Append text to the paragraph TextRange textRange1 = paragraph.appendText("Java's"); TextRange textRange2 = paragraph.appendText(" versatility and wide range of applications make it "); TextRange textRange3 = paragraph.appendText("one of the most popular programming languages"); TextRange textRange4 = paragraph.appendText(" in the world. Its strong community support and continuous evolution " + "ensure it remains relevant in modern software development."); // Create a ParagraphStyle object ParagraphStyle style = new ParagraphStyle(document); style.setName("MyStyle"); style.getCharacterFormat().setBold(false); style.getCharacterFormat().setTextColor(Color.black); style.getCharacterFormat().setFontName("Times New Roman"); style.getCharacterFormat().setFontSize(13f); // Add the style the document document.getStyles().add(style); // Apply the style to the paragraph paragraph.applyStyle(style.getName()); // Change the font style of the specified text ranges textRange1.getCharacterFormat().setTextColor(Color.blue); textRange3.getCharacterFormat().setItalic(true); textRange3.getCharacterFormat().setTextColor(Color.red); // Change the font name and size if you want // textRange1.getCharacterFormat().setFontName("Arial"); // textRange1.getCharacterFormat().setFontSize(15f); // Set the horizontal alignment paragraph.getFormat().setHorizontalAlignment(HorizontalAlignment.Left); // Save the document to a docx file document.saveToFile("output/ApplyMultipleFonts.docx", FileFormat.Docx_2019); // Dispose resources document.dispose(); } }
Use a Private Font in a Word Document in Java
In creative fields like graphic design and marketing, incorporating private fonts in a Word document can enhance aesthetics, making it more engaging and visually unique. To add a custom font to a document, use the Document.getPrivateFontList().add() method. To ensure the document displays correctly on systems without the font installed, embed the font using the Document.setEmbedFontsInFile() method.
The steps to incorporate private fonts in a Word document are as follows:
- Create a Document object.
- Load a Word file, and add a paragraph to it.
- Create a PrivateFontPath object, specifying the name and path of a private font.
- Add the font to document using the Document.getPrivateFontList().add() method.
- Embed fonts in the generated file by passing true to the Document.setEmbedFontsInFile() method.
- Apply the font to the paragraph using the TextRange.getCharacterFormat().setFontName() method.
- Save the document to a different Word file.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.PrivateFontPath; import com.spire.doc.Section; import com.spire.doc.documents.Paragraph; import com.spire.doc.fields.TextRange; public class ApplyPrivateFont { public static void main(String[] args) { // Create a Document instance Document document = new Document(); // Load a Word document document.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx"); // Get the last section Section section = document.getLastSection(); // Add a paragraph Paragraph paragraph = section.addParagraph(); // Append text to the paragraph TextRange textRange = paragraph.appendText("Java's versatility and wide range of applications make it one of the most popular " + "programming languages in the world. Its strong community support and continuous evolution ensure " + "it remains relevant in modern software development."); // Create a PrivateFontPath object, specifying font name and path PrivateFontPath fontPath = new PrivateFontPath(); fontPath.setFontName("Otto"); fontPath.setFontPath("C:\\Users\\Administrator\\Desktop\\Otto.ttf"); // Add the private font to the document document.getPrivateFontList().add(fontPath); // Embed fonts in the generated file document.setEmbedFontsInFile(true); // Apply font to text range textRange.getCharacterFormat().setFontName("Otto"); textRange.getCharacterFormat().setFontSize(28f); // Save the document to file document.saveToFile("output/ApplyPrivateFont.docx", FileFormat.Docx); // Dispose resources document.dispose(); } }
Change the Font of the Specified Text in Java
Changing the font of specific text can highlight key information, enhancing its visibility for readers. Spire.Doc for Java offers the Document.findAllString() method, allowing developers to search for a specified string within an existing document. Once the text is located, you can assign it to a TextRange object, which provides various APIs for formatting the text.
The steps to change the of the specified text in a Word document using Java are as follows:
- Create a Document object.
- Load a Word file.
- Find all the occurrences of the specified string using the Document.findAllString() method.
- Iterate through the occurrences.
- Get the specific occurrence (TextSection) as a TextRange object.
- Get the CharacterFormat object using the TextRange.getCharacterFormat() method.
- Change the font name, style, size and text color of the text range using the methods under the CharacterFormat object.
- Save the document to a different Word file.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.documents.TextSelection; import com.spire.doc.documents.UnderlineStyle; import com.spire.doc.fields.TextRange; import java.awt.*; public class ChangeFontOfText { public static void main(String[] args) { // Create a Document instance Document document = new Document(); // Load a Word document document.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx"); // Find the text you wish to modify the font for TextSelection[] textSelections = document.findAllString("Rich Standard Library", false, true); // Iterate through the find results for (int i = 0; i < textSelections.length; i++) { // Get a specific text selection as a text range TextRange textRange = textSelections[i].getAsOneRange(); // Change the text color and style textRange.getCharacterFormat().setTextColor(Color.blue); textRange.getCharacterFormat().setUnderlineStyle(UnderlineStyle.Dash); textRange.getCharacterFormat().setBold(true); // Change font name and size if you want // textRange.getCharacterFormat().setFontName("Calibri"); // textRange.getCharacterFormat().setFontSize(18f); } // Save the result document document.saveToFile("output/ChangeFontOfText.docx", FileFormat.Docx_2019); // Dispose resources document.dispose(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
In certain circumstances, you may need to insert superscripts and subscripts in Microsoft Word. For instance, when you are creating an academic document involving scientific formulas. In this article, you will learn how to insert superscripts and subscripts into Word documents in Java using Spire.Doc for Java library.
Install Spire.Doc for Java
First, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>13.6.2</version> </dependency> </dependencies>
Insert Superscripts and Subscripts into Word using Java
The following are the main steps to insert a superscript or subscript into a Word document using Spire.Doc for Java:
- Create a Document instance.
- Load a Word document using Document.loadFromFile() method.
- Get the specific section using Document.getSections().get(sectionIndex) method.
- Add a paragraph to the section using Section.addParagraph() method.
- Add normal text to the paragraph using Paragraph.appendText() method.
- Add superscript or subscript text to the paragraph using Paragraph.appendText() method.
- Apply superscript or subscript formatting to the superscript or subscript text through TextRange.getCharacterFormat().setSubSuperScript() method.
- Save the result document using Document.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.Section; import com.spire.doc.documents.BreakType; import com.spire.doc.documents.Paragraph; import com.spire.doc.documents.SubSuperScript; import com.spire.doc.fields.TextRange; public class InsertSuperscriptAndSubscript { public static void main(String[] args){ //Create a Document instance Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Get the first section Section section = document.getSections().get(0); //Add a paragraph to the section Paragraph paragraph = section.addParagraph(); //Add normal text to the paragraph paragraph.appendText("E = mc"); //Add superscript text to the paragraph TextRange superscriptText = paragraph.appendText("2"); //Apply superscript formatting to the superscript text superscriptText.getCharacterFormat().setSubSuperScript(SubSuperScript.Super_Script); //Start a new line paragraph.appendBreak(BreakType.Line_Break); //Add normal text to the paragraph paragraph.appendText("H"); //Add subscript text to the paragraph TextRange subscriptText = paragraph.appendText("2"); //Apply subscript formatting to the subscript text subscriptText.getCharacterFormat().setSubSuperScript(SubSuperScript.Sub_Script); //Add normal text to the paragraph paragraph.appendText("O"); //Set font size for the text in the paragraph for(Object item : paragraph.getItems()) { if (item instanceof TextRange) { TextRange textRange = (TextRange)item ; textRange.getCharacterFormat().setFontSize(36f); } } //Save the result document document.saveToFile("InsertSuperscriptAndSubscript.docx", FileFormat.Docx_2013); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Changing the font color of a certain paragraph or text can help you make the paragraph or text stand out in your Word document. In this article, we will demonstrate how to change the font color in Word in Java using Spire.Doc for Java library.
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>13.6.2</version> </dependency> </dependencies>
Change Font Color of a Paragraph in Java
The following are the steps to change the font color of a paragraph in a Word document:
- Create a Document instance.
- Load the Word document using Document.LoadFromFile() method.
- Get the desired section using Document.getSections().get(sectionIndex) method.
- Get the desired paragraph that you want to change the font color of using Section.getParagraphs().get(paragraphIndex) method.
- Create a ParagraphStyle instance.
- Set the style name and font color using ParagraphStyle.setName() and ParagraphStyle.getCharacterFormat().setTextColor() methods.
- Add the style to the document using Document.getStyles().add() method.
- Apply the style to the paragraph using Paragraph.applyStyle() method.
- Save the result document using Document.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.Section; import com.spire.doc.documents.Paragraph; import com.spire.doc.documents.ParagraphStyle; import java.awt.*; public class ChangeFontColorForParagraph { public static void main(String []args){ //Create a Document instance Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Get the first section Section section = document.getSections().get(0); //Change text color of the first Paragraph Paragraph p1 = section.getParagraphs().get(0); ParagraphStyle s1 = new ParagraphStyle(document); s1.setName("Color1"); s1.getCharacterFormat().setTextColor(new Color(188, 143, 143)); document.getStyles().add(s1); p1.applyStyle(s1.getName()); //Change text color of the second Paragraph Paragraph p2 = section.getParagraphs().get(1); ParagraphStyle s2 = new ParagraphStyle(document); s2.setName("Color2"); s2.getCharacterFormat().setTextColor(new Color(0, 0, 139));; document.getStyles().add(s2); p2.applyStyle(s2.getName()); //Save the result document document.saveToFile("ChangeParagraphTextColor.docx", FileFormat.Docx); } }
Change Font Color of a Specific Text in Java
The following are the steps to change the font color of a specific text in a Word document:
- Create a Document instance.
- Load a Word document using Document.loadFromFile() method.
- Find the text that you want to change font color of using Document.findAllString() method.
- Loop through all occurrences of the searched text and change the font color for each occurrence using TextSelection.getAsOneRange().getCharacterFormat().setTextColor() method.
- Save the result document using Document.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.documents.TextSelection; import java.awt.*; public class ChangeFontColorForText { public static void main(String []args){ //Create a Document instance Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Find the text that you want to change font color for TextSelection[] text = document.findAllString("Spire.Doc for .NET", false, true); //Change the font color for the searched text for (TextSelection seletion : text) { seletion.getAsOneRange().getCharacterFormat().setTextColor(Color.red); } //Save the result document document.saveToFile("ChangeCertainTextColor.docx", FileFormat.Docx); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Embedding and applying private fonts in Word or PDF documents can make your documents unique. This article demonstrates how to embed fonts in a Word document as well as a converted PDF document, by using Spire.Doc for Java.
Embed Font in Word Document
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.PrivateFontPath; import com.spire.doc.documents.Paragraph; import com.spire.doc.fields.TextRange; public class EmbedPrivateFontInWord { public static void main(String[] args) { //create a Word document Document document = new Document(false);
//add a paragraph Paragraph paragraph = document.addSection().addParagraph(); //embed a private font in Word document document.setEmbedFontsInFile(true); PrivateFontPath fontPath = new PrivateFontPath(); fontPath.setFontName("Otto"); fontPath.setFontPath("C:\\Users\\Administrator\\Desktop\\Otto.ttf"); document.getPrivateFontList().add(fontPath); //add text to the paragraph and apply the embedded font TextRange tr = paragraph.appendText("Spire.Doc for Java is a professional Java Word API that enables "+ "Java applications to create, convert, manipulate and print Word documents without " + "using Microsoft Office."); tr.getCharacterFormat().setFontName("Otto"); tr.getCharacterFormat().setFontSize(26f); //save to file document.saveToFile("output/EmbedFont.docx", FileFormat.Docx); } }
Embed Font in Converted PDF Document
import com.spire.doc.Document; import com.spire.doc.PrivateFontPath; import com.spire.doc.ToPdfParameterList; import com.spire.doc.documents.Paragraph; import com.spire.doc.fields.TextRange; import java.util.*; public class EmbedPrivateFontInConvertedPDF { public static void main(String[] args) { //create a Word document Document document = new Document(false);
//add a paragraph Paragraph paragraph = document.addSection().addParagraph(); //initialize PrivateFontPath object PrivateFontPath fontPath = new PrivateFontPath("Otto","C:\\Users\\Administrator\\Desktop\\Otto.ttf"); //add text to paragraph and apply the private font TextRange tr = paragraph.appendText("Spire.Doc for Java is a professional Java Word API that enables "+ "Java applications to create, convert, manipulate and print Word documents without " + "using Microsoft Office."); tr.getCharacterFormat().setFontName("Otto"); tr.getCharacterFormat().setFontSize(26f); //create a ToPdfParameterList object ToPdfParameterList toPdfParameterList = new ToPdfParameterList(); //set private fonts as a parameter for converting Word to PDF List pathList = new LinkedList<>(); pathList.add(fontPath); toPdfParameterList.setPrivateFontPaths(pathList); //save to PDF file document.saveToFile("output/EmbedFont.pdf",toPdfParameterList); } }
