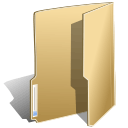
Conversion (22)
Combining multiple images into a single PDF document is an efficient method for those who want to store or distribute their images in a more organized way. Converting these images into a single PDF file not only saves storage space but also ensures that all images are kept together in one place, making it easier and more convenient to share or transfer them. In this article, you will learn how to merge several images into a single PDF document in Java using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert Multiple Images into a Single PDF Document in Java
In order to convert all the images in a folder to a PDF, we iterate through each image, add a new page to the PDF with the same size as the image, and then draw the image onto the new page. The following are the detailed steps.
- Create a PdfDocument object.
- Set the page margins to zero using PdfDocument.getPageSettings().setMargins() method.
- Get the folder where the images are stored.
- Iterate through each image file in the folder, and get the width and height of a specific image.
- Add a new page that has the same width and height as the image to the PDF document using PdfDocument.getPages().add() method.
- Draw the image on the page using PdfPageBase.getCanvas().drawImage() method.
- Save the document using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.graphics.PdfImage; import java.awt.*; import java.io.File; public class ConvertMultipleImagesIntoPdf { public static void main(String[] args) { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Set the page margins to 0 doc.getPageSettings().setMargins(0); //Get the folder where the images are stored File folder = new File("C:/Users/Administrator/Desktop/Images"); //Iterate through the files in the folder for (File file : folder.listFiles()) { //Load a particular image PdfImage pdfImage = PdfImage.fromFile(file.getPath()); //Get the image width and height int width = pdfImage.getWidth(); int height = pdfImage.getHeight(); //Add a page that has the same size as the image PdfPageBase page = doc.getPages().add(new Dimension(width, height)); //Draw image at (0, 0) of the page page.getCanvas().drawImage(pdfImage, 0, 0, width, height); } //Save to file doc.saveToFile("CombineImagesToPdf.pdf"); doc.dispose(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Converting a PDF document to an image format like JPEG or PNG can be useful for a variety of reasons, such as making it easier to share the content on social media, embedding it into a website, or including it in a presentation. Converting PDF to images can also avoid printing issues caused by the printers that do not support PDF format well enough. In this article, you will learn how to convert PDF to JPEG or PNG in Java using Spire.PDF for Java.
Install Spire.PDF for Java
First, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert PDF to JPEG in Java
The PdfDocument.saveAsImage() method provided by Spire.PDF for Java enables the conversion of a particular page from a PDF document into a BufferedImage object, which can be saved as a file in .jpg or .png format. The following are the steps to convert each page of a PDF document to a JPEG image file.
- Create a PdfDocument object.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Iterate through each page of the PDF document.
- Convert a specific page into a BufferedImage object using PdfDocument.saveAsImage() method.
- Re-create a BufferedImage in RGB type with the same width and height as the converted image.
- Write the image data as a .jpg file using ImageIO.write() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.graphics.PdfImageType; import javax.imageio.ImageIO; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; public class ConvertPdfToJpeg { public static void main(String[] args) throws IOException { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a sample PDF document pdf.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); //Loop through the pages for (int i = 0; i < pdf.getPages().getCount(); i++) { //Save the current page as a buffered image BufferedImage image = pdf.saveAsImage(i, PdfImageType.Bitmap, 300, 300); //Re-create a buffered image in RGB type BufferedImage newImg = new BufferedImage(image.getWidth(), image.getHeight(), BufferedImage.TYPE_INT_RGB); newImg.getGraphics().drawImage(image, 0, 0, null); //Write the image data as a .jpg file File file = new File("C:\\Users\\Administrator\\Desktop\\Output\\" + String.format(("ToImage-%d.jpg"), i)); ImageIO.write(newImg, "JPEG", file); } pdf.close(); } }
Convert PDF to PNG in Java
The steps to convert PDF to PNG using Spire.PDF for Java are as follows.
- Create a PdfDocument object.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Iterate through each page of the PDF document.
- Convert a specific page into a BufferedImage object using PdfDocument.saveAsImage() method.
- Write the image data as a .png file using ImageIO.write() method.
- Java
import com.spire.pdf.*; import javax.imageio.ImageIO; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; public class ConvertPdfToPng { public static void main(String[] args) throws IOException { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Load a sample PDF document doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); //Make the background of the generated PNG files transparent //doc.getConvertOptions().setPdfToImageOptions(0); //Loop through the pages for (int i = 0; i < doc.getPages().getCount(); i++) { //Save the current page as a buffered image BufferedImage image = doc.saveAsImage(i); //Write the image data as a .png file File file = new File("C:\\Users\\Administrator\\Desktop\\Output\\" + String.format("ToImage-%d.png", i)); ImageIO.write(image, "png", file); } doc.close(); } }
If the background of the PDF document is white and you want to make it transparent when converted to PNG, you can add following line of code before you save each page to a BufferedImage object.
- Java
doc.getConvertOptions().setPdfToImageOptions(0);
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF is a popular and widely used file format, but when it comes to giving presentations to others in meetings, classes or other scenarios, PowerPoint is always preferred over PDF files because it contains rich presentation effects that can better capture the attention of your audience. In this article, you will learn how to convert an existing PDF file to a PowerPoint presentation using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert PDF to PowerPoint Presentation in Java
From Version 9.2.1, Spire.PDF for Java supports converting PDF to PPTX using PdfDocument.saveToFile() method. With this method, each page of your PDF file will be converted to a single slide in PowerPoint. Below are the steps to convert a PDF file to an editable PowerPoint document.
- Create a PdfDocument instance.
- Load a sample PDF document using PdfDocument.loadFromFile() method.
- Save the document as a PowerPoint document using PdfDocument.saveToFile(String filename, FileFormat.PPTX) method.
- Java
import com.spire.pdf.FileFormat; import com.spire.pdf.PdfDocument; public class PDFtoPowerPoint { public static void main(String[] args) { //Create a PdfDocument instance PdfDocument pdfDocument = new PdfDocument(); //Load a sample PDF document pdfDocument.loadFromFile("sample.pdf"); //Convert PDF to PPTX document pdfDocument.saveToFile("PDFtoPowerPoint.pptx", FileFormat.PPTX); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Text files can be easily edited by any text editing program. If you want to prevent changes when others view the files, you can convert them to PDF. In this article, we will demonstrate how to convert text files to PDF in Java using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert Text Files to PDF in Java
The following are the main steps to convert a text file to PDF using Spire.PDF for Java:
- Read the text in the text file into a String object.
- Create a PdfDocument instance and add a page to the PDF file using PdfDocument.getPages().add() method.
- Create a PdfTextWidget instance from the text.
- Draw the text onto the PDF page using PdfTextWidget.draw() method.
- Save the result file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.FileFormat; import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.graphics.*; import java.awt.geom.Rectangle2D; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class ConvertTextToPdf { public static void main(String[] args) throws Exception { //Read the text from the text file String text = readTextFromFile("Input.txt"); //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Add a page PdfPageBase page = pdf.getPages().add(); //Create a PdfFont instance PdfFont font = new PdfFont(PdfFontFamily.Helvetica, 11); //Create a PdfTextLayout instance PdfTextLayout textLayout = new PdfTextLayout(); textLayout.setBreak(PdfLayoutBreakType.Fit_Page); textLayout.setLayout(PdfLayoutType.Paginate); //Create a PdfStringFormat instance PdfStringFormat format = new PdfStringFormat(); format.setLineSpacing(20f); //Create a PdfTextWidget instance from the text PdfTextWidget textWidget = new PdfTextWidget(text, font, PdfBrushes.getBlack()); //Set string format textWidget.setStringFormat(format); //Draw the text at the specified location of the page Rectangle2D.Float bounds = new Rectangle2D.Float(); bounds.setRect(0,25,page.getCanvas().getClientSize().getWidth(),page.getCanvas().getClientSize().getHeight()); textWidget.draw(page, bounds, textLayout); //Save the result file pdf.saveToFile("TextToPdf.pdf", FileFormat.PDF); } public static String readTextFromFile(String fileName) throws IOException { StringBuffer sb = new StringBuffer(); BufferedReader br = new BufferedReader(new FileReader(fileName)); String content = null; while ((content = br.readLine()) != null) { sb.append(content); sb.append("\n"); } return sb.toString(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
The Tagged Image File Format (TIFF) is a relatively flexible image format which has the advantages of not requiring specific hardware, as well as being portable. Spire.PDF for Java supports converting TIFF to PDF and vice versa. This article will show you how to programmatically convert PDF to TIFF using it from the two aspects below.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert All Pages of a PDF File to TIFF
The following steps show you how to convert all pages of a PDF file to a TIFF file.
- Create a PdfDocument instance.
- Load a PDF sample document using PdfDocument.loadFromFile() method.
- Save all pages of the document to a TIFF file using PdfDocument.saveToTiff(String tiffFilename) method.
- Java
import com.spire.compression.TiffCompressionTypes; import com.spire.pdf.PdfDocument; public class PDFToTIFF { public static void main(String[] args) { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a PDF sample document pdf.loadFromFile("sample.pdf"); //Save all pages of the document to Tiff pdf.saveToTiff("output/PDFtoTiff.tiff"); } }
Convert Some Specified Pages of a PDF File to TIFF
The following steps are to convert specified pages of a PDF document to a TIFF file.
- Create a PdfDocument instance.
- Load a PDF sample document using PdfDocument.loadFromFile() method.
- Save specified pages of the document to a TIFF file using PdfDocument.saveToTiff(String tiffFilename, int startPage, int endPage, int dpix, int dpiy) method.
- Java
import com.spire.pdf.PdfDocument; public class PDFToTIFF { public static void main(String[] args) { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a PDF sample document pdf.loadFromFile("sample.pdf"); //Save specified pages of the document to TIFF and set horizontal and vertical resolution pdf.saveToTiff("output/ToTiff2.tiff",0,1,400,600); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
TIFF or TIF files are image files saved in the tagged image file format. They are often used for storing high-quality color images. Sometimes, you may wish to convert TIFF files to PDF so you can share them more easily. In this article, you will learn how to achieve this task programmatically in Java using Spire.PDF for Java library.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert TIFF to PDF in Java
The following are the steps to convert a TIFF file to PDF:
- Create an instance of PdfDocument class.
- Add a page to the PDF using PdfDocument.getPages().add() method.
- Create a PdfImage object from the TIFF image using PdfImage.fromFile() method.
- Draw the image onto the page using PdfPageBase.getCanvas().drawImage() method.
- Save the result file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.FileFormat; import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.graphics.PdfImage; public class ConvertTiffToPdf { public static void main(String[] args){ //Create a PdfDocument instance PdfDocument doc = new PdfDocument(); //Add a page PdfPageBase page = doc.getPages().add(); //Create a PdfImage object from the TIFF image PdfImage tiff = PdfImage.fromFile("Input.tiff"); //Draw the image to the page page.getCanvas().drawImage(tiff, 20, 0, 450, 450); //Save the result file doc.saveToFile("TiffToPdf.pdf", FileFormat.PDF); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Spire.PDF for Java offers the PdfDocument.saveToFile() method to convert PDF to other file formats such as Word, Excel, HTML, SVG and XPS. When converting PDF to Excel, it allows you to convert each PDF page to a single Excel worksheet or convert multiple PDF pages to one Excel worksheet. This article will demonstrate how to convert a PDF file containing 3 pages to one Excel worksheet.
Install Spire.PDF for Java
First of all, you're required to add the Spire.PDF.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert a Multi-page PDF to One Excel Worksheet
The detailed steps are as follows:
- Create a PdfDocument object.
- Load a sample PDF file using PdfDocument.loadFromFile() method.
- Set the PDF to XLSX conversion options to render multiple PDF pages on a single worksheet using PdfDocument.getConvertOptions().setPdfToXlsxOptions() method.
- Save the PDF file to Excel using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; import com.spire.pdf.conversion.XlsxLineLayoutOptions; public class ManyPagesToOneSheet { public static void main(String[] args) { //Create a PdfDocument object PdfDocument pdf = new PdfDocument(); //Load a sample PDF file pdf.loadFromFile("C:\\Users\\Administrator\\Desktop\\Members.pdf"); //Set the PDF to XLSX conversion options: rendering multiple pages on a single worksheet pdf.getConvertOptions().setPdfToXlsxOptions(new XlsxLineLayoutOptions(false,true,true)); //Save to Excel pdf.saveToFile("out/ToOneSheet.xlsx", FileFormat.XLSX); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Postscript, also known as PS, is a dynamically typed, concatenative programming language that describes the appearance of a printed page. Owing to its faster printing and improved quality, sometime you may need to convert a PDF document to Postscript. In this article, you will learn how to achieve this function using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.PDF.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert PDF to PostScript
The following are the steps to convert PDF to PostScript.
- Create a PdfDocument object.
- Load a sample PDF file using PdfDocument.loadFromFile() method.
- Save the document as PostScript using PdfDocument.saveToFile() method
- Java
import com.spire.pdf.*; public class PDFToPS { public static void main(String[] args) { //Load a pdf document PdfDocument doc = new PdfDocument(); doc.loadFromFile("sample.pdf"); //Convert to PostScript file doc.saveToFile("output.ps", FileFormat.POSTSCRIPT); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Spire.PDF for Java offers PdfDocument.saveAsImage() method to convert PDF document to image. From Version 4.11.1, Spire.PDF for Java supports to set the transparent value for the background of the resulted images during PDF to image conversion. This article will show you how to convert PDF to images with transparent background in Java applications.
Install Spire.PDF for Java
First of all, you need to add the Spire.PDF.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>11.6.2</version> </dependency> </dependencies>
Convert PDF to Images with Transparent Background
- Create an object of PdfDocument class.
- Load a sample PDF document using PdfDocument.loadFromFile() method.
- Specify the transparent value for the background of the resulted images using PdfDocument.getConvertOptions().setPdfToImageOptions() method.
- Save the document to images using PdfDocument.saveAsImage() method.
- Java
import com.spire.pdf.*; import javax.imageio.ImageIO; import java.awt.image.BufferedImage; import java.io.File; public class PdftoImage { public static void main(String[] args) throws Exception { //Create an object of PdfDocument class. PdfDocument pdf = new PdfDocument(); //Load the sample PDF document pdf.loadFromFile("Sample.pdf"); //Specify the background transparent value as 0 during PDF to image conversion. pdf.getConvertOptions().setPdfToImageOptions(0); //Save PDF to .png image BufferedImage image = pdf.saveAsImage(0); File file = new File( String.format("ToImage.png")); ImageIO.write(image, "PNG", file); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
We have demonstrated how to use Spire.PDF for java to convert PDF to image. This article will show you how to convert image to PDF in Java applications.
import com.spire.pdf.*; import com.spire.pdf.graphics.PdfImage; public class imageToPDF { public static void main(String[] args) throws Exception { //Create a PDF document PdfDocument pdf = new PdfDocument(); //Add a new page PdfPageBase page = pdf.getPages().add(); //Load the image PdfImage image = PdfImage.fromFile("logo.jpg"); double widthFitRate = image.getPhysicalDimension().getWidth() / page.getCanvas().getClientSize().getWidth(); double heightFitRate = image.getPhysicalDimension().getHeight() / page.getCanvas().getClientSize().getHeight(); double fitRate = Math.max(widthFitRate, heightFitRate); //get the picture width and height double fitWidth = image.getPhysicalDimension().getWidth() / fitRate; double fitHeight = image.getPhysicalDimension().getHeight() / fitRate; //Draw image page.getCanvas().drawImage(image, 0, 30, fitWidth, fitHeight); // Save document to file pdf.saveToFile("output/ToPDF.pdf"); pdf.close(); } }
Output:
